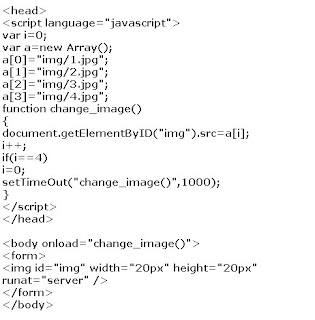
Search This Blog
15 February 2011
10 February 2011
GridView Insertion
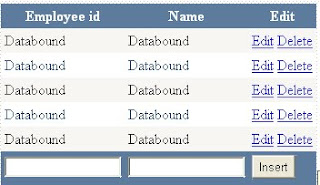
// coding in gridview.aspx source file //
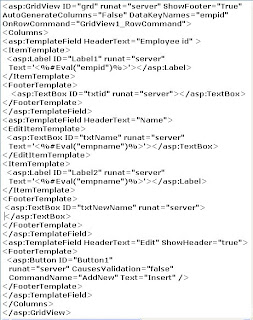
protected void GridView1_RowCommand(object sender, GridViewCommandEventArgs e)
{
if (e.CommandName.Equals("AddNew"))
{
TextBox txtNewName = (TextBox)grd.FooterRow.FindControl("txtNewName");
TextBox t1 = (TextBox)grd.FooterRow.FindControl("txtid");
c.cmd.CommandText = "select * from emp";
c.adp.Fill(c.ds, "st");
c.dr = c.ds.Tables["st"].NewRow();
c.dr["empid"] = t1.Text;
c.dr["empname"] = txtNewName.Text;
c.ds.Tables["st"].Rows.Add(c.dr);
c.scb = new System.Data.SqlClient.SqlCommandBuilder(c.adp);
c.adp.Update(c.ds.Tables["st"]);
bind();
}
}
09 February 2011
Gridview edit,delete,cancel.paging
// coding in gridview.aspx source file //
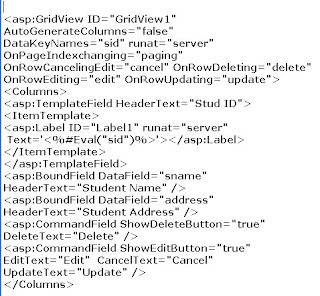
// coding in gridview.aspx.cs file //
public partial class admin_manageAttendance : System.Web.UI.Page
{
connection c = new connection();
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
bind();
}
}
public void bind()
{
c.cmd.commandText="Select * from student";
c.adp.Fill(c.ds,"vt");
GridView1.DataSource=c.ds.Tables["vt"];
GridView1.DataBind();
}
protected void delete(object sender, GridViewDeleteEventArgs e)
{
c.con.Open();
c.cmd.CommandText = "delete from student where sid='"+GridView1.DataKeys[e.RowIndex].Value.ToString()+"'";
c.cmd.ExecuteNonQuery();
c.con.Close();
GridView1.EditIndex = -1;
bind();
}
protected void cancel(object sender, GridViewCancelEditEventArgs e)
{
GridView1.EditIndex = -1;
bind();
}
protected void edit(object sender, GridViewEditEventArgs e)
{
GridView1.EditIndex = e.NewEditIndex;
bind();
}
protected void update(object sender, GridViewUpdateEventArgs e)
{
Label l=new Label();
TextBox t1 = new TextBox();
TextBox t2 = new TextBox();
GridViewRow gr= GridView1.Rows[e.RowIndex];
t1 = (TextBox)gr.Cells[1].Controls[0];
t2 = (TextBox)gr.Cells[2].Control[0];
c.con.Open();
c.cmd.CommandText = "update student set sname='"+ t1.Text+ "', address='"+ t2.Text+"' where sid='" + GridView1.DataKeys[e.RowIndex].Value.ToString() + "'";
c.cmd.ExecuteNonQuery();
c.con.Close();
GridView1.EditIndex = -1;
bind();
}
protected void paging(object sender, GridViewPageEventArgs e)
{
GridView1.PageIndex = e.NewPageIndex;
bind();
}
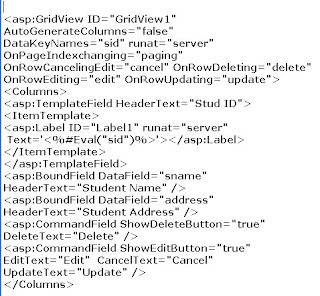
public partial class admin_manageAttendance : System.Web.UI.Page
{
connection c = new connection();
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
bind();
}
}
public void bind()
{
c.cmd.commandText="Select * from student";
c.adp.Fill(c.ds,"vt");
GridView1.DataSource=c.ds.Tables["vt"];
GridView1.DataBind();
}
protected void delete(object sender, GridViewDeleteEventArgs e)
{
c.con.Open();
c.cmd.CommandText = "delete from student where sid='"+GridView1.DataKeys[e.RowIndex].Value.ToString()+"'";
c.cmd.ExecuteNonQuery();
c.con.Close();
GridView1.EditIndex = -1;
bind();
}
protected void cancel(object sender, GridViewCancelEditEventArgs e)
{
GridView1.EditIndex = -1;
bind();
}
protected void edit(object sender, GridViewEditEventArgs e)
{
GridView1.EditIndex = e.NewEditIndex;
bind();
}
protected void update(object sender, GridViewUpdateEventArgs e)
{
Label l=new Label();
TextBox t1 = new TextBox();
TextBox t2 = new TextBox();
GridViewRow gr= GridView1.Rows[e.RowIndex];
t1 = (TextBox)gr.Cells[1].Controls[0];
t2 = (TextBox)gr.Cells[2].Control[0];
c.con.Open();
c.cmd.CommandText = "update student set sname='"+ t1.Text+ "', address='"+ t2.Text+"' where sid='" + GridView1.DataKeys[e.RowIndex].Value.ToString() + "'";
c.cmd.ExecuteNonQuery();
c.con.Close();
GridView1.EditIndex = -1;
bind();
}
protected void paging(object sender, GridViewPageEventArgs e)
{
GridView1.PageIndex = e.NewPageIndex;
bind();
}
Globally Detailview Binding
public void detail_bind(DetailView dv, string query)
{
ds.Clear();
cmd.CommandText = query;
adp.Fill(ds, "vtt");
dv.DataSource = ds.Tables["vtt"];
dv.DataBind();
}
}
// How to call this global function in managecourse.aspx.cs file //
public partial class managecourse : System.Web.UI.Page
{
connection c = new connection();
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
c.detail_bind(DetailView1, "select * from student where sid='"+Session["sname"].ToString()+"'");
}
}
}
{
ds.Clear();
cmd.CommandText = query;
adp.Fill(ds, "vtt");
dv.DataSource = ds.Tables["vtt"];
dv.DataBind();
}
}
// How to call this global function in managecourse.aspx.cs file //
public partial class managecourse : System.Web.UI.Page
{
connection c = new connection();
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
c.detail_bind(DetailView1, "select * from student where sid='"+Session["sname"].ToString()+"'");
}
}
}
Globally Gridview Binding
public void bindgrid(GridView gv, string query)
{
ds.Clear();
cmd.CommandText = query;
adp.Fill(ds, "vt1");
gv.DataSource = ds.Tables["vt1"];
gv.DataBind();
}
}
// How to call this global function in managecourse.aspx.cs file //
public partial class managecourse : System.Web.UI.Page
{
connection c = new connection();
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
c.bindgrid(GridView1, "select * from student");
}
}
}
{
ds.Clear();
cmd.CommandText = query;
adp.Fill(ds, "vt1");
gv.DataSource = ds.Tables["vt1"];
gv.DataBind();
}
}
// How to call this global function in managecourse.aspx.cs file //
public partial class managecourse : System.Web.UI.Page
{
connection c = new connection();
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
c.bindgrid(GridView1, "select * from student");
}
}
}
Globally Dropdownlist Binding
create this function in global class file(Class1.cs)-
public void bindddl(DropDownList ddlid, string query, string tf, string vf)
{
ds.Clear();
cmd.CommandText = query;
adp.Fill(ds, "vt");
ddlid.DataSource = ds.Tables["vt"];
ddlid.DataTextField = tf;
ddlid.DataValueField = vf;
ddlid.DataBind();
}
// Calling of this function //
public partial class managecourse : System.Web.UI.Page
{
connection c = new connection();
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
c.bindddl(ddlcourse,"select * from course_master","course_name","course_id");
ddlcourse.Items.Insert(0,"Select course");
}
}
}
public void bindddl(DropDownList ddlid, string query, string tf, string vf)
{
ds.Clear();
cmd.CommandText = query;
adp.Fill(ds, "vt");
ddlid.DataSource = ds.Tables["vt"];
ddlid.DataTextField = tf;
ddlid.DataValueField = vf;
ddlid.DataBind();
}
// Calling of this function //
public partial class managecourse : System.Web.UI.Page
{
connection c = new connection();
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
c.bindddl(ddlcourse,"select * from course_master","course_name","course_id");
ddlcourse.Items.Insert(0,"Select course");
}
}
}
Global Connection Class
First you will have to create a class file under App_Code Folder.
add SqlClient namespace as given below-
using System.Data.SqlClient;
public class connection
{
public SqlConnection con = new SqlConnection();
public SqlCommand cmd = new SqlCommand();
public SqlDataAdapter adp = new SqlDataAdapter();
public SqlCommandBuilder scb = new SqlCommandBuilder();
public DataSet ds = new DataSet();
public DataRow dr;
public connection()
{
con.ConnectionString = "Data Source=.\\sqlexpress;User Id=sa;Password=pass; Database=testdb";
cmd.Connection = con;
adp.SelectCommand = cmd;
}
add SqlClient namespace as given below-
using System.Data.SqlClient;
public class connection
{
public SqlConnection con = new SqlConnection();
public SqlCommand cmd = new SqlCommand();
public SqlDataAdapter adp = new SqlDataAdapter();
public SqlCommandBuilder scb = new SqlCommandBuilder();
public DataSet ds = new DataSet();
public DataRow dr;
public connection()
{
con.ConnectionString = "Data Source=.\\sqlexpress;User Id=sa;Password=pass; Database=testdb";
cmd.Connection = con;
adp.SelectCommand = cmd;
}
Subscribe to:
Posts (Atom)